How to Create a Responsive Table with HTML and CSS
Author
Pushpendra Singh
Date Published
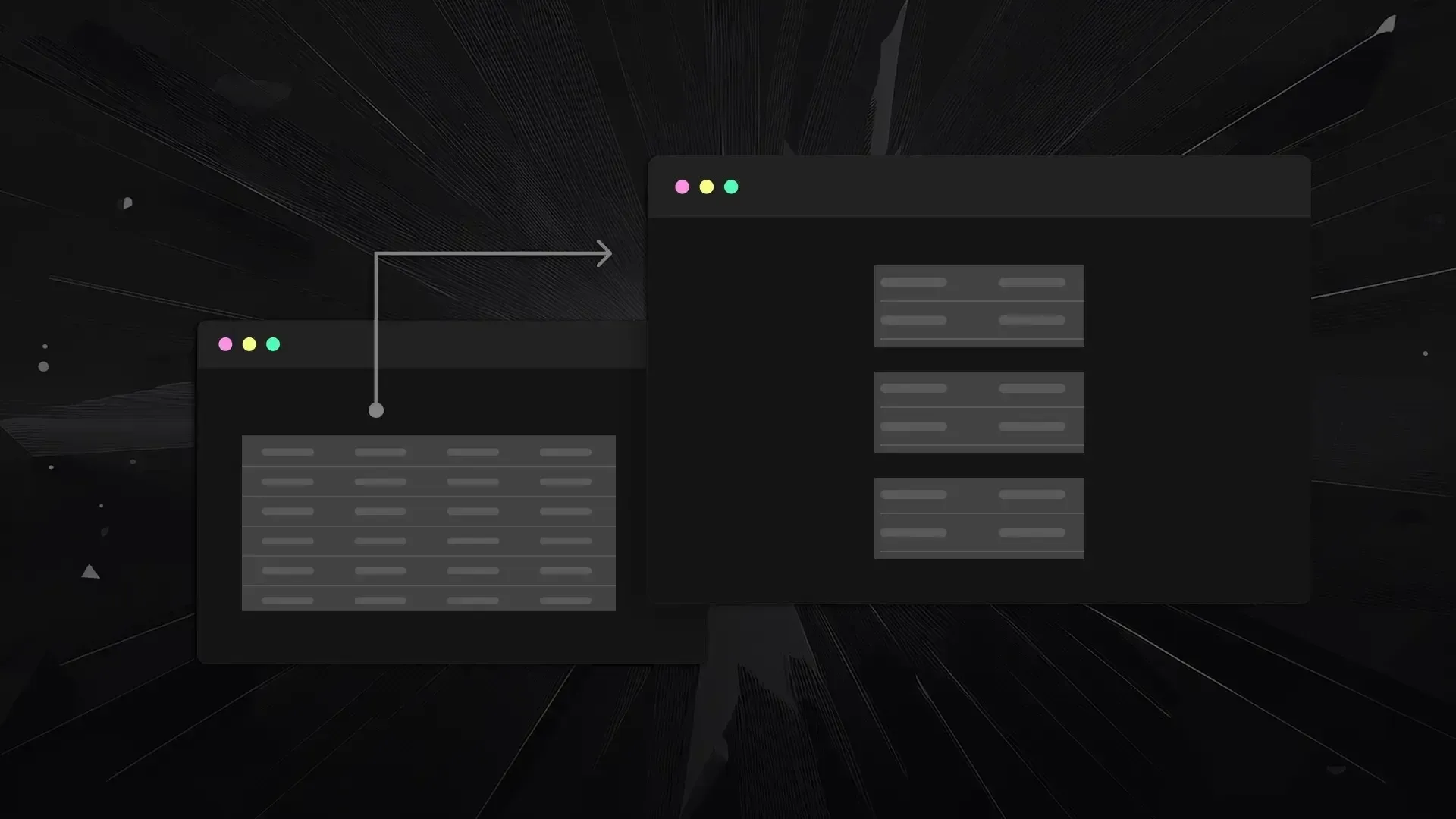
In this tutorial, we'll learn how to build a mobile-responsive table using HTML and CSS without relying on JavaScript or external libraries.
HTML Structure
We start by building a simple table layout, where each column has a heading and some data rows. The table contains four columns: "Sr. No.", "First Name", "Last Name," and "Handle." Here's the structure:
1<!DOCTYPE html>2<html>3<head>4 <title>Responsive Table Tutorial</title>5 <meta name="viewport" content="width=device-width, initial-scale=1">6 <link rel="stylesheet" href="./style.css" />7</head>8<body>9 <h1>Responsive Table Example</h1>10 <table class="table">11 <thead>12 <tr>13 <th>Sr. No.</th>14 <th>First Name</th>15 <th>Last Name</th>16 <th>Handle</th>17 </tr>18 </thead>19 <tbody>20 <tr>21 <th data-title="Sr. No.">1</th>22 <td data-title="First Name">Mark</td>23 <td data-title="Last Name">Otto</td>24 <td data-title="Handle">@mdo</td>25 </tr>26 <tr>27 <th data-title="Sr. No.">2</th>28 <td data-title="First Name">Jacob</td>29 <td data-title="Last Name">Thornton</td>30 <td data-title="Handle">@fat</td>31 </tr>32 <tr>33 <th data-title="Sr. No.">3</th>34 <td data-title="First Name">Larry</td>35 <td data-title="Last Name">Bird</td>36 <td data-title="Handle">@twitter</td>37 </tr>38 </tbody>39 </table>40</body>41</html>42
Here is the CSS for Responsiveness
To make the table responsive, especially for mobile devices, we use a media query that hides the table header and displays each cell as a block with labels for each column. This makes the table adaptable to smaller screens.
1/* General Styles */2h1 {3 text-align: center;4 margin-top: 40px;5 color: #05395E;6}78table {9 width: 100%;10 max-width: 720px;11 margin: 40px auto;12 box-shadow: 0px 4px 20px rgba(5,57,94,0.5);13}1415/* Mobile styles */16@media screen and (max-width: 767px) {17 table thead {18 display: none;19 }20 table tbody, table tr {21 display: block;22 width: 100%;23 }24 table tr th::before, table tr td::before {25 content: attr(data-title);26 display: block;27 font-weight: bold;28 }29 table tr th, table tr td {30 display: block;31 width: 50%;32 float: left;33 }34 table tr {35 margin-bottom: 16px;36 clear: both;37 }38}39
Conclusion
1. Media Queries: This CSS uses a media query to apply styles for devices smaller than 767px.
2. Data Attributes: The data-title attribute displays the table headers in the mobile view.
3. Float and Block Display: Table cells are displayed as blocks and floated for better stacking on small screens.
By doing this the table looks good on both desktop and mobile devices.
Feel free to adjust the design according to your needs. If you want to dive deeper into this responsive table design, watch out the full video tutorial published on YouTube by CodiesBugs.