How to configure Jest with Next.js for Unit Testing
Author
Abhishek Chaubey
Date Published
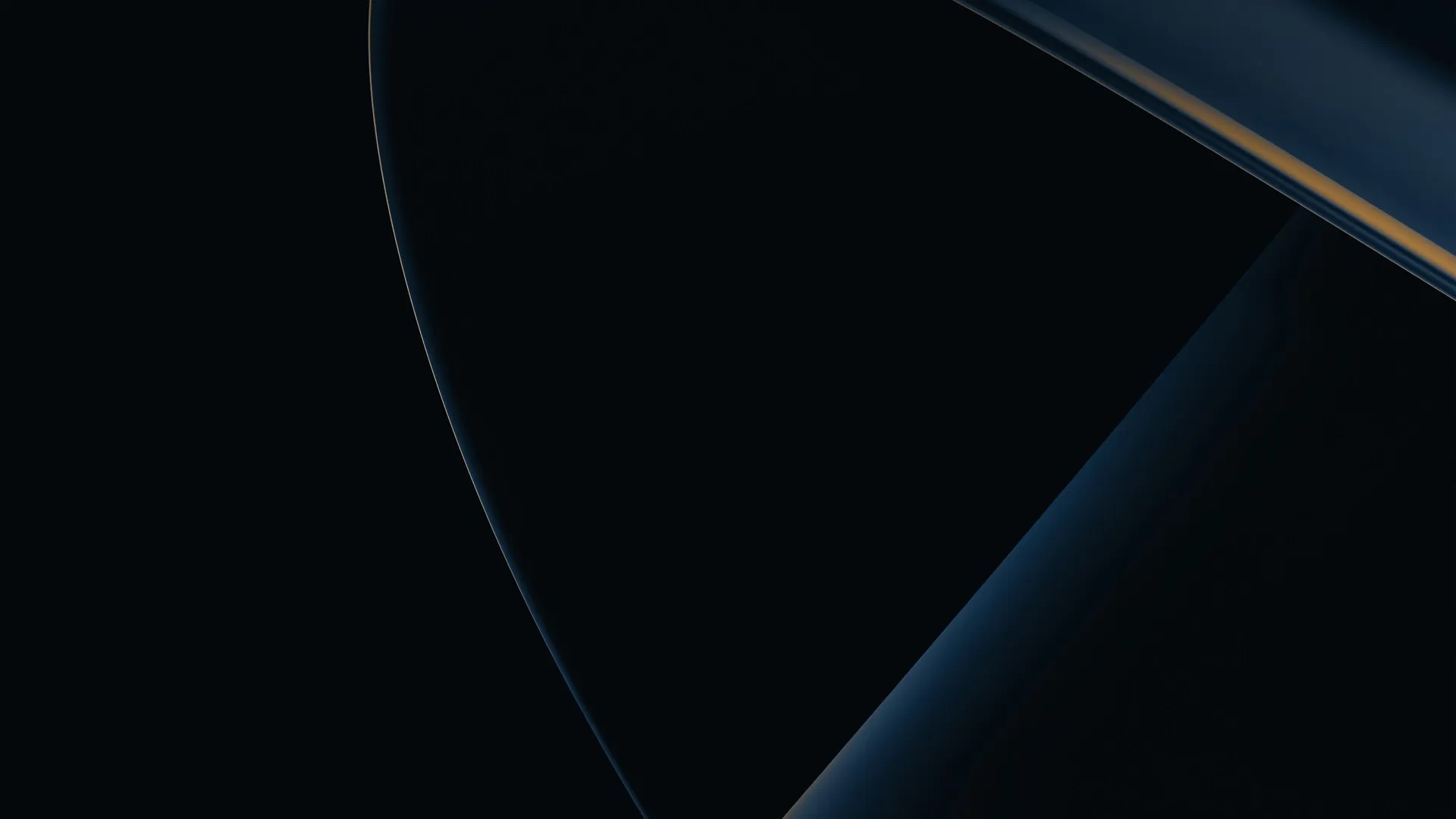
When working with Next.jsew, testing is an essential part of the development process to ensure your app functions as expected. Jest is a powerful JavaScript testing framework that's widely used for unit tests, integration tests, and snapshot testing. In this article, we will cover how to set up Jest in a Next.js project and provide some example test cases, including how to use snapshot testing.
Step 1: Install Jest and Required Dependencies
To set up Jest with Next.js, we need to install Jest and some additional packages. In your Next.js project, run the following command:
1npm install --save-dev jest @testing-library/react @testing-library/jest-dom @testing-library/user-event babel-jest
jest: The main testing framework.
@testing-library/react: Helps in testing React components.
@testing-library/jest-dom: Provides custom matchers for Jest that work with the DOM.
@testing-library/user-event: Simulates user events like typing, clicking, etc.
babel-jest: Enables Babel to compile tests written in ES6/ES7 syntax.
Step 2: Update the package.json File
Add the following Jest configuration to your package.json file to integrate it with Next.js:
12{3 "jest": {4 "testEnvironment": "jsdom",5 "setupFilesAfterEnv": ["<rootDir>/jest.setup.js"],6 "moduleNameMapper": {7 "\\.(css|scss|sass)$": "identity-obj-proxy",8 "^@/components/(.*)$": "<rootDir>/components/$1"9 }10 }11}1213
testEnvironment: Specifies jsdom as the environment, which simulates a browser-like environment in Node.js for testing React components.
setupFilesAfterEnv: Specifies a setup file (jest.setup.js) that will be executed before each test.
moduleNameMapper: Maps CSS imports and aliases used in your project (e.g., @/components).
Step 3: Create jest.setup.js
Create a new file called jest.setup.js at the root of your project and add the following code:
12import '@testing-library/jest-dom/extend-expect';
This imports jest-dom's custom matchers like .toHaveTextContent() or .toBeInTheDocument() for improved assertions when working with DOM elements.
Step 4: Example Test Case
Let’s write a simple test case for a Next.js component. Suppose we have a component called Button.tsx inside a components/ folder.
1// components/Button.tsx2import React from 'react';34const Button = ({ label }: { label: string }) => {5 return <button>{label}</button>;6};78export default Button;9
Now, we create a test file Button.test.tsx in the same directory as Button.tsx.
12// components/Button.test.tsx3import { render, screen } from '@testing-library/react';4import Button from './Button';56describe('Button component', () => {7 it('renders with the correct label', () => {8 render(<Button label="Click Me" />);9 const buttonElement = screen.getByText(/Click Me/i);10 expect(buttonElement).toBeInTheDocument();11 });12});13
This test case renders the Button component with the label "Click Me" and checks whether the button element is in the document.
Step 5: Snapshot Testing
Snapshot testing is useful for ensuring that the UI doesn’t change unexpectedly. Let’s extend the Button component test with a snapshot test.
12// components/Button.test.tsx3import { render } from '@testing-library/react';4import Button from './Button';56describe('Button component', () => {7 it('matches the snapshot', () => {8 const { asFragment } = render(<Button label="Click Me" />);9 expect(asFragment()).toMatchSnapshot();10 });11});12
This test creates a snapshot of the component's rendered output. When the test is run for the first time, Jest saves a snapshot file. On subsequent runs, Jest compares the component output with the saved snapshot to check for changes.
Step 6: Run Tests
1"scripts": {2 "test": "jest"3}
Now, you can run your tests using:
1npm run test
Conclusion
By following the steps outlined in this article, you can easily configure Jest for testing in your Next.js project. With features like DOM testing, user event simulation, and snapshot testing, Jest makes it easy to test your components thoroughly. You can now confidently write tests and ensure that your Next.js application behaves as expected!
References
The guide provides additional details and configuration steps for integrating Jest seamlessly with Next.js projects. You can find it at the following link:
https://nextjs.org/docs/app/building-your-application/testing/jest